实用的 JavaScript 一行代码
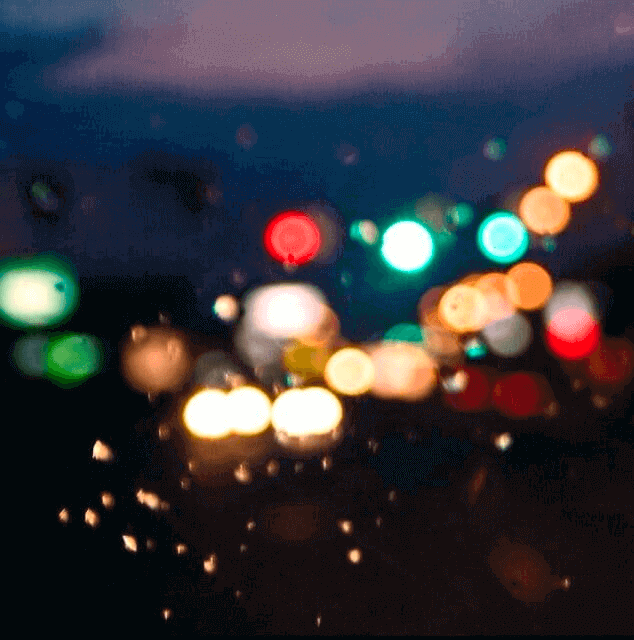
检查日期是否有效
1 | const isDateValid = (...val) => !Number.isNaN(new Date(...val).valueOf()) |
计算两个日期之间的间隔天数
1 | const dayDif = (date1, date2) => Math.ceil(Math.abs(date1.getTime() - date2.getTime()) / 86400000) |
查找日期位于一年中的第几天
1 | const dayOfYear = (date) => Math.floor((date - new Date(date.getFullYear(), 0, 0)) / 1000 / 60 / 60 / 24) |
字符串首字母大写
1 | const capitalize = str => str.charAt(0).toUpperCase() + str.slice(1) |
翻转字符串
1 | const reverseStr = str => str.split('').reverse().join('') |
生成一个随机字符串
1 | const randomString = () => Math.random().toString(36).slice(2) |
生成一个 UUID 字符串
1 | const getUuid = () => Number(Math.random().toString().substr(2, 5) + Date.now()).toString(36) |
从指定位置截断字符串
1 | const truncateString = (string, length) => string.length < length ? string : `${string.slice(0, length)}...` |
去除字符串中的 HTML 标签
1 | const stripHtml = html => (new DOMParser().parseFromString(html, 'text/html')).body.textContent || '' |
去除数组中的重复项
1 | const myUnique = array => [...new Set(array)] |
获取一组数字的平均值
1 | const average = (...args) => args.reduce((a, b) => a + b) / args.length |
获取指定位数四舍五入的值
1 | const myRound = (n, d) => Number(Math.round(n + "e" + d) + "e-" + d) |
RGB 转化为十六进制
1 | const rgbToHex = (r, g, b) => "#" + ((1 << 24) + (r << 16) + (g << 8) + b).toString(16).slice(1) |
获取选中的文本
1 | const getSelectedText = () => window.getSelection().toString() |
检测是否是黑暗模式
1 | const isDarkMode = window.matchMedia && window.matchMedia('(prefers-color-scheme: dark)').matches |
获取变量的类型
1 | const myTypeOf = (data) => Object.prototype.toString.call(data).slice(8, -1).toLowerCase() |
判断对象是否为空
1 | const isEmptyObj = obj => Reflect.ownKeys(obj).length === 0 && obj.constructor === Object |
持续完善中 …
Comments
Comment plugin failed to load
Loading comment plugin