实用的 JavaScript 字符串内置方法
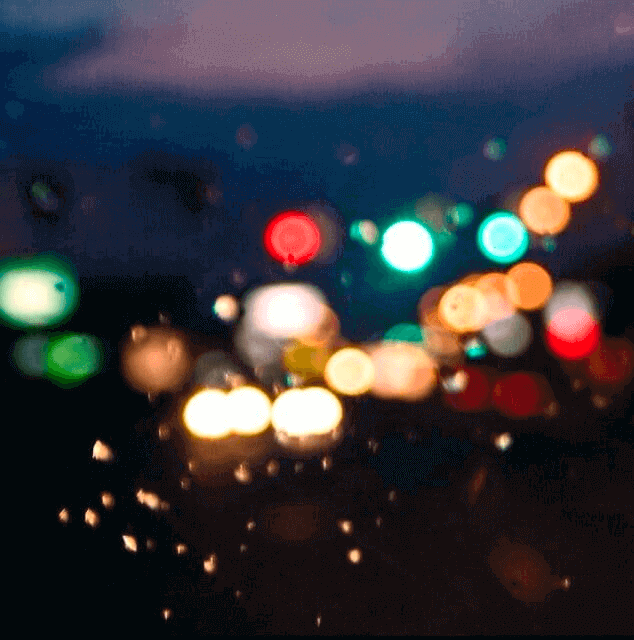
在 JavaScript 中,字符串是一种常用的数据类型,作为我们开发人员使用最频繁的数据类型之一,本文介绍一些你可能不太了解但又非常实用的字符串内置方法,帮助你提升开发效率,快速完成数据处理。
charAt(index)
返回指定索引位置的字符。
1 | const str = 'Hello, World' |
charCodeAt(index)
返回指定索引位置字符的 Unicode 编码。
1 | const str = 'Hello, World' |
concat(str1, str2, …)
连接两个或多个字符串。
1 | const str1 = 'Hello' |
indexOf(searchValue, startIndex)
返回指定字符串在原字符串中首次出现的位置索引。
1 | const str = 'Hello, World' |
lastIndexOf(searchValue, startIndex)
返回指定字符串在原字符串中最后一次出现的位置索引。
1 | const str = 'Hello, World' |
slice(startIndex, endIndex)
提取字符串的一部分,返回新字符串。
1 | const str = 'Hello, World' |
substring(startIndex, endIndex)
与 slice
类似,提取字符串的一部分,返回新字符串。
1 | const str = 'Hello, World' |
substr(startIndex, length)
提取字符串的一部分,返回新字符串,基于起始位置和长度。
1 | const str = 'Hello, World' |
toUpperCase()
将字符串转换为大写。
1 | const str = 'Hello, World' |
toLowerCase()
将字符串转换为小写。
1 | const str = 'Hello, World' |
replace(searchValue, replaceValue)
替换字符串中的指定值。
1 | const str = 'Hello, World' |
split(separator, limit)
将字符串拆分为子字符串数组。
1 | const str = 'apple,orange,banana' |
trim()
去除字符串两端的空格。
1 | const str = ' Hello, World ' |
Comments
Comment plugin failed to load
Loading comment plugin